Indicators
Indicators represent a single value that is computed from measurements within the specified time window and are calculated using an operator.
There are three operations for calculating indicators:
- Average - The average of all measurements within the time window
- Count - The count of all measurements within the time window
- Rate - The error rate of all measurements within the time window
Create an indicator
To create an indicator, navigate to the application indicators page and click the Create Indicator button.
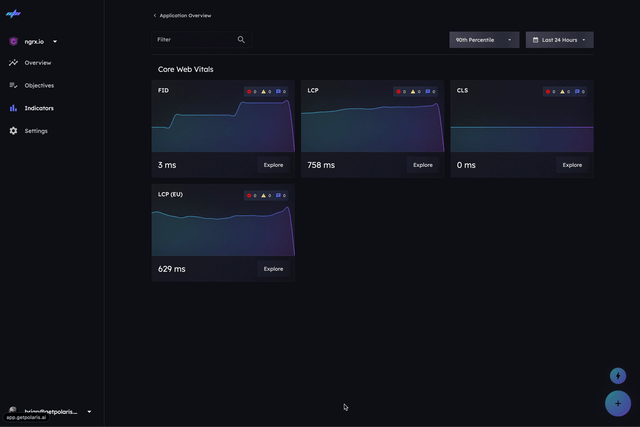
Next, specify a name for the indicator.
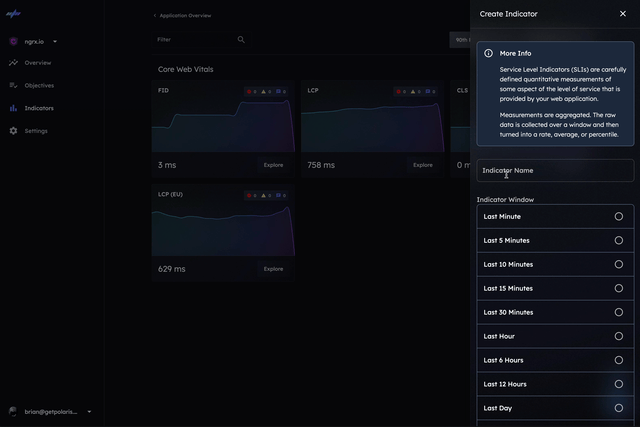
Next, choose the indicator window.
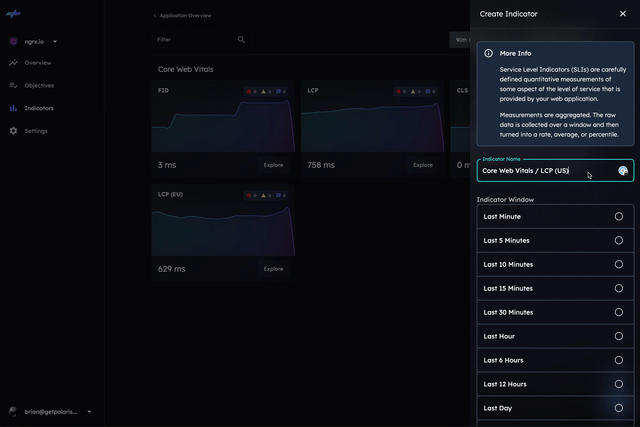
Next, choose the indicator operation.
- Average: The average value of all measurements in the window.
- Rate: The error rate of all measurements in the window.
- Count: The number of measurements in the window.
Finally, we filter all measurements that our application is sending to Polaris using a simple predicate function. We'll cover this is more detail next.
Indicator Measurements
The measurements used to calculate an indicator are determined by the provided predicate function.
A feature of Polaris is not having to learn a new query language to filter measurements. Instead, Polaris uses a predicate function that is written in JavaScript. This allows you to use the full power of JavaScript to filter measurements.
Predicate Function
First, let's look at the anatomy of a predicate function.
{
// return true if the measurement should be included in the indicator
// return false if the measurement should be excluded from the indicator
}
A few things to note:
- First, the function must be named
main
. - Second, the function must accept a single argument,
measurement
. - Third, the function must return a boolean value.
The indicator predicate function is invoked with the Measurement
object.
interface Measurement {
id: string;
appId: string;
eventName: string;
duration: number;
result: MeasurementResult;
timestamp: DateTime;
connectionQuality: ConnectionQuality | null;
deviceType: DeviceType;
location: Location | null;
userMetadata: object;
}
Learn more about the Measurement
object
Filter by the Measurement Event Name
The most common predicate functions simply filter measurement by the event name. The event name is the unique string specified for a measurement when instrumenting your application.
function main(measurement) {
return measurement.event === 'my-event-name';
}
Filter by user location
The measurement
data is enriched with the user's location that is determined via IP-address reverse lookup.
We can use this location data to filter measurements for an indicator.
First, here is an example predicate function of an indicator for users in the United States.
function main(measurement) {
return measurement.eventName = 'auth-flow'
&& measurement.location.country === 'US';
}
Next, here is an example predicate function of an indicator for users in New York City.
function main(measurement) {
return measurement.eventName = 'auth-flow'
&& measurement.location.country === 'US'
&& measurement.location.region === 'New York'
&& measurement.location.city === 'New York';
}
Filter by user device type
Here is an example predicate function of an indicator for users in New York City that are using a phone.
function main(measurement) {
return measurement.eventName = 'auth-flow'
&& measurement.location.country === 'US'
&& measurement.location.region === 'New York'
&& measurement.location.city === 'New York'
&& measurement.deviceType === 'PHONE';
}
Filter by user connection quality
Here is an example predicate function of an indicator for users in New York City that are using a phone and have a fast (4g equivalent) connection quality.
function main(measurement) {
return measurement.eventName = 'auth-flow'
&& measurement.location.country === 'US'
&& measurement.location.region === 'New York'
&& measurement.location.city === 'New York'
&& measurement.deviceType === 'PHONE'
&& measurement.connectionQuality === 'FAST';
}
Filter by user metadata
You can also filter measurements by user metadata.
Here is an example predicate function of an indicator for users in New York City that are using a phone and have a fast (4g equivalent) connection quality and have a premium
user metadata key set to true
.
function main(measurement) {
return measurement.eventName = 'auth-flow'
&& measurement.location.country === 'US'
&& measurement.location.region === 'New York'
&& measurement.location.city === 'New York'
&& measurement.deviceType === 'PHONE'
&& measurement.connectionQuality === 'FAST'
&& measurement.userMetadata.premium === true;
}