Let's get started
- Install and configure the Polaris SDK.
- Instrument your app to send measurements to Polaris.
- Create indicators of performance and reliability.
- Create goal-based objectives.
- Configure webhooks to receive notifications when objective thresholds are exceeded.
Install the Polaris SDK
System requirements:
- Node.js 18 or later
- macOS, Windows (including WSL), and Linux are supported.
npm i -S @getpolaris.ai/sdk @getpolaris.ai/sdk-react
Configure the SDK
To configure the SDK, you will need your app’s API Key. You can find the API Key by going to your app in Polaris and clicking on Settings.
<PolarisProvider apiKey={import.meta.env.VITE_POLARIS_API_KEY}>
<Outlet />
</PolarisProvider>
Measure Render Performance
Measure the render performance of your React application.
Use the PolarisProfiler
component to measure the mount and update phases of your components.
import { PolarisProfiler } from '@getpolaris.ai/sdk-react';
export function App() {
return (
<>
<PolarisProfiler id="nav">
<Navigation />
</PolarisProfiler>
<PolarisProfiler id="table">
<DataTable />
</PolarisProfiler>
</>
);
}
Measure Critical Workflows
Measure the performance of critical workflows in your application.
First, use the useInstrument
hook to create an instrument.
Invoke the start()
method to start the measurement timer.
In the event of an exception, invoke the fail()
method.
import { useInstrument } from '@getpolaris.ai/sdk-react';
export function Authenticate() {
const instrument = useInstrument('auth-flow');
const handleSubmit = useCallback((email, password) => {
try {
instrument.start();
} catch (error) {
instrument.fail({ error });
}
}, []);
return (
<div>...</div>
);
}
Then, get a reference to the instrument using the useInstrument
hook.
Invoke the stop()
method to stop the measurement timer.
import { useInstrument } from '@getpolaris.ai/sdk-react';
export function Reviews() {
const instrument = useInstrument('auth-flow');
useEffect(() => {
getReviews()
.then((reviews) => {
instrument.done();
})
.catch((error) => {
instrument.fail();
});
}, []);
return (
<div>...</div>
);
}
Create an indicator
An indicator is a metric that you want to track. For example, you might want to track the success/failure rate of an API request. Or, you might want to track core web vitals for your web application.
Core Web Vitals
Note: Core web vitals measurements are automatically sent to Polaris once you connect your application to Polaris.
To create an indicator, navigate to the application indicators page and click the Create Indicator button.
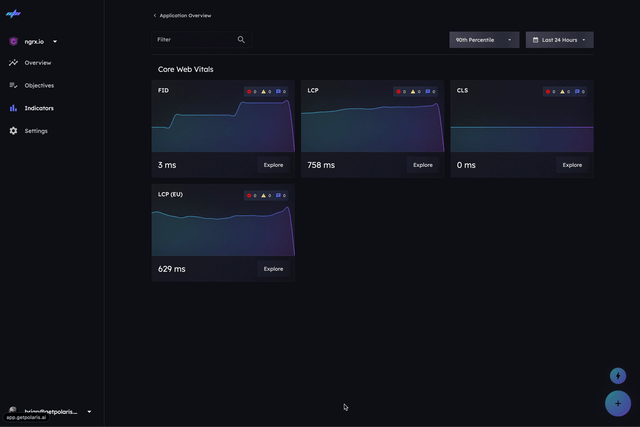
Next, specify a name for the indicator.
Naming Convention
Note: You can use a forward slash (/
) to create a hierarchy of indicators.
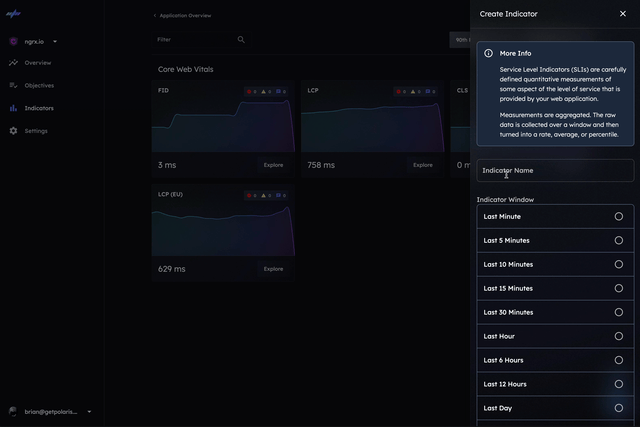
Next, choose the indicator window.
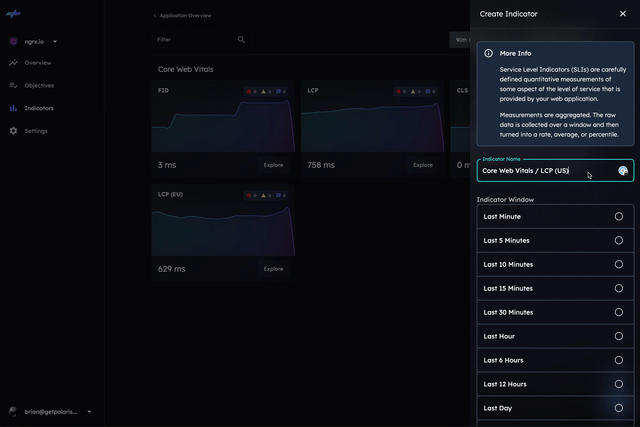
Next, choose the indicator operation.
- Average: The average value of all measurements in the window.
- Rate: The error rate of all measurements in the window.
- Count: The number of measurements in the window.
Finally, we filter all measurements that our application is sending to Polaris using a simple predicate function.
In this example, we'll filter all measurements with the eventName
of lcp
.
function main(measurement) {
return measurement.eventName === 'lcp';
}
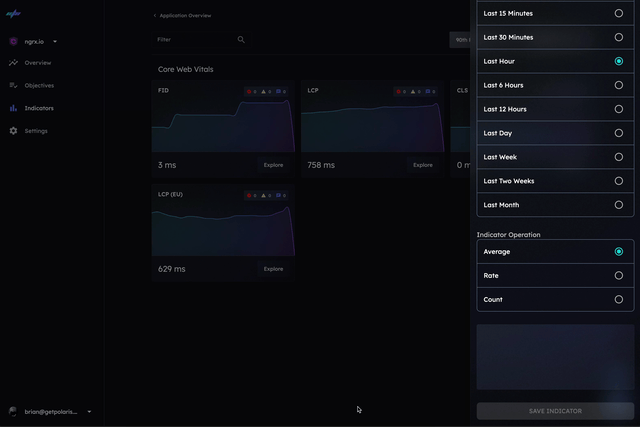
Create an objective
To create an objective, navigate to the application objectives page and click the Create Objective button.
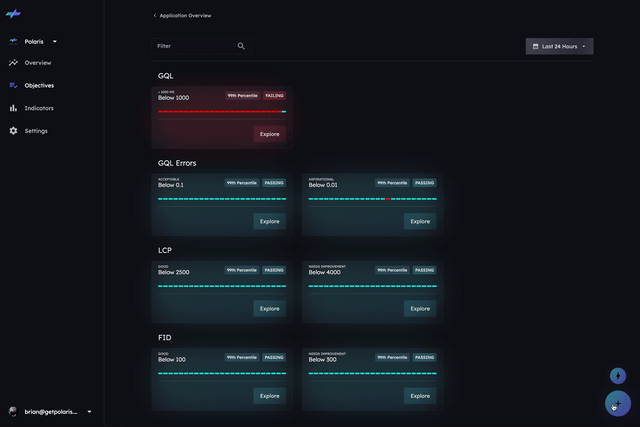
Next, specify a name for the objective.
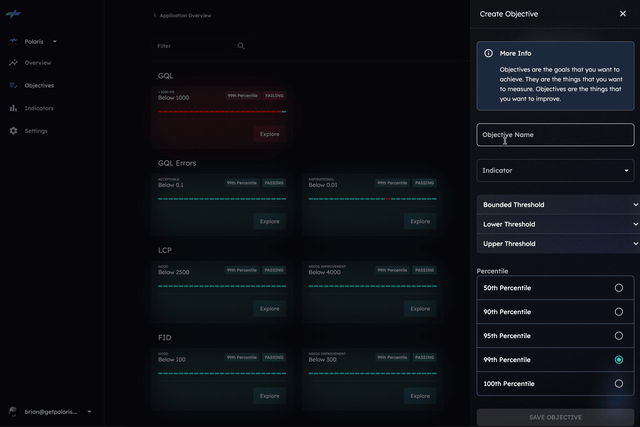
Next, choose the indicator we just created.
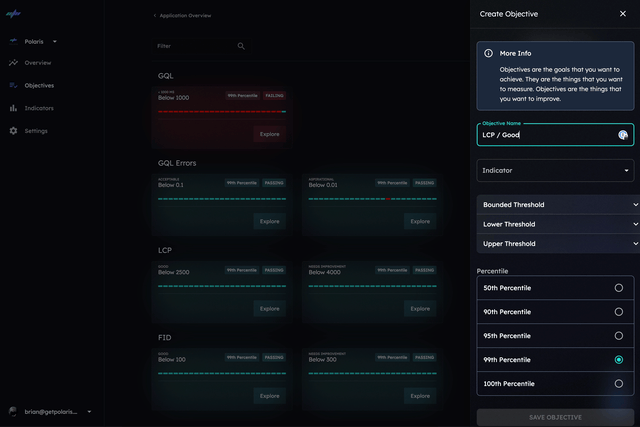
Next, choose the appropriate threshold for the objective.
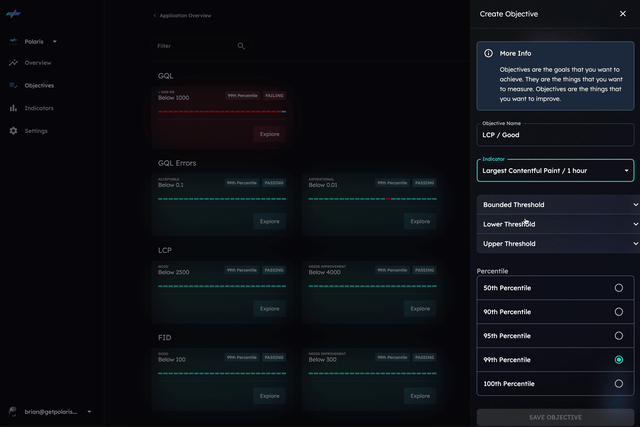
Finally, choose the percentile for the objective.
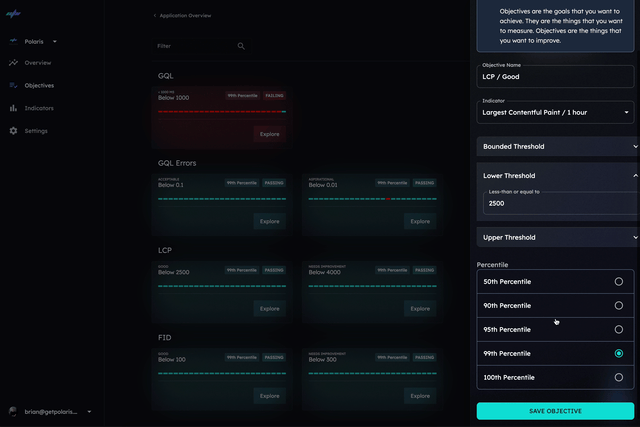
Webhooks
Configure one or more webhooks to be notified when an objective state changes.
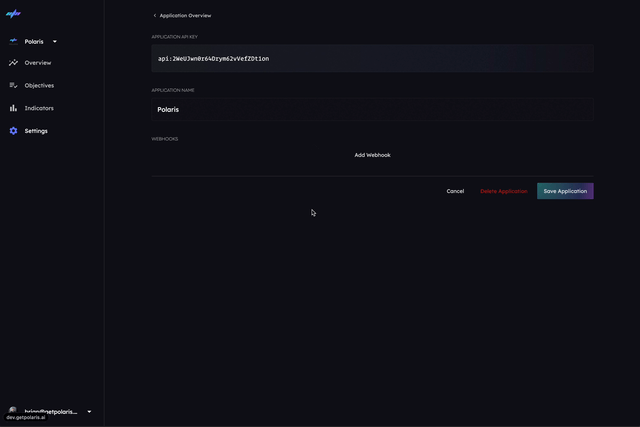
The webhook is a POST request to the URL you specify. The body of the request is a JSON object with the following structure:
{
"event": "ObjectiveStateChange",
"objective": {
"id": "2WeUJuj8uImopO9qhgbTZxhuDGM",
"appId": "2WfoQifyOYe6wmM6fAwFLZvlxep";
"name": "Auth / Login / Acceptible";
"state": "FAILING";
"stateLastUpdated": "2023-12-10T14:16:05.755Z",
"createdAt": "2023-01-01T00:00:00Z",
"updatedAd": "2023-01-01T00:00:00Z"
},
"state": "FAILING",
"app": {
"id": "app-id",
"name": "app-name",
"description": "app-description"
}
"indicator": {
"id": "2WeUJuj8uImopO9qhgbTZxhuDGM",
"appId": "indicator-name",
"name": "Auth / Login"
"window": "LAST_HOUR"
"operation": "AVERATE"
"createdAt": "2023-01-01T00:00:00Z",
"updatedAd": "2023-01-01T00:00:00Z"
}
}
What's next?
Congrats - you're now streaming real-time measurements into Polaris, you have established indicators of performance and reliability and the objectives that you and your team desire to achieve. 🎉